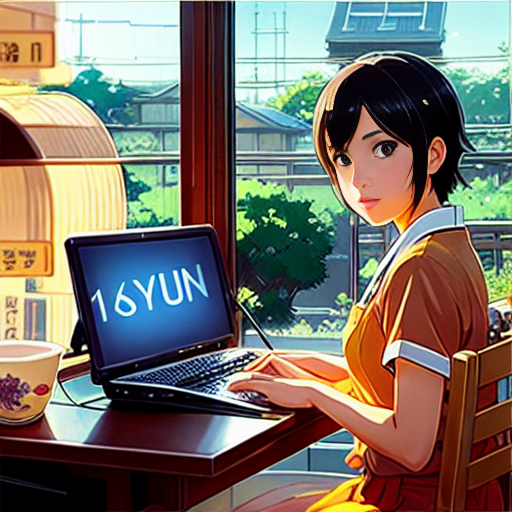
亿牛云代理
HTMLAgilityPack是一款备受欢迎的用于解析和操作HTML文档的库。在使用之前,开发者需要考虑一些优缺点。下面是一些值得注意的优点:
- 强大的错误容忍性:HTMLAgilityPack可以处理其他解析器可能拒绝或无法解析的格式错误或无效的HTML文档。
- 灵活的API:它提供了一个灵活而强大的API,使开发者能够使用XPath、LINQ或CSS选择器来查询和修改HTML节点,满足不同的需求。
- 广泛的应用场景:HTMLAgilityPack支持.NET Framework和.NET Core,可用于各种场景,包括网页抓取、数据提取和HTML清理等。
然而,也有一些缺点需要考虑:
- 性能问题:处理大型或复杂的HTML文档时,特别是在使用XPath查询时,HTMLAgilityPack可能会遇到一些性能问题。
- 对最新HTML特性的支持限制:HTMLAgilityPack可能不支持一些最新的HTML特性或标准,例如HTML5或SVG。
- 可能存在依赖和冲突:在使用HTMLAgilityPack时,可能会引入一些依赖或与其他使用HTMLAgilityPack的库或框架发生冲突的情况。
下面以采集https://www.booking.com网站的酒店及评价为示例:
代码语言:c#
复制
using System; using System.IO; using System.Net; using System.Net.Http; using HtmlAgilityPack;
class Program
{
static async Task Main(string[] args)
{
// 亿牛云(动态转发隧道代理)
//爬虫代理加强版 代理服务器的认证信息
string proxyUrl = "www.16yun.cn";
string proxyPort = "3100";
string proxyUsername = "16YUN";
string proxyPassword = "16IP";// 目标网站的URL string url = "https://www.booking.com"; // 创建HttpClientHandler以设置代理认证 HttpClientHandler handler = new HttpClientHandler { Proxy = new WebProxy(proxyUrl,proxyPort), UseProxy = true, PreAuthenticate = true, UseDefaultCredentials = false, Credentials = new NetworkCredential(proxyUsername, proxyPassword) }; // 创建HttpClient,并设置代理 HttpClient client = new HttpClient(handler); // 发送HTTP GET请求并获取网页内容 HttpResponseMessage response = await client.GetAsync(url); response.EnsureSuccessStatusCode(); string html = await response.Content.ReadAsStringAsync(); // 使用HTMLAgilityPack解析HTML文档 HtmlDocument document = new HtmlDocument(); document.LoadHtml(html); // 从HTML中提取酒店名字和评价信息 var hotelNames = document.DocumentNode.SelectNodes("//h3[contains(@class, 'sr-hotel__name')]"); var hotelRatings = document.DocumentNode.SelectNodes("//div[contains(@class, 'bui-review-score__badge')]"); // 创建CSV文件 using (StreamWriter writer = new StreamWriter("hotels.csv")) { writer.WriteLine("Hotel Name, Rating"); // 将数据写入CSV文件 for (int i = 0; i < hotelNames.Count; i++) { string name = hotelNames[i].InnerText.Trim(); string rating = hotelRatings[i].InnerText.Trim(); writer.WriteLine($"{name}, {rating}"); } } Console.WriteLine("数据已成功抓取并保存为CSV文件。"); }
}
上述程序运行后,将抓取https://www.booking.com网站上的酒店名字和评价,并将其保存为名为"hotels.csv"的CSV文件。在CSV文件中,每一行包含酒店名字和对应的评价。